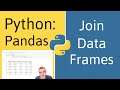
How to Join Data Frames in Pandas (Python)
↓ Code Available Below! ↓
This video shows how to join pandas data frames using the merge function. Joining data that resides in different tables is a common data preparation task when creating an analytical data set from multiple sources. The merge function allows you to perform inner, outer, left and right joins by changing the how argument.
If you find this video useful, like, share and subscribe to support the channel!
► Subscribe: https://www.youtube.com/c/DataDaft?sub_confirmation=1
Code used in this Python Code Clip:
import pandas as pd
data1 = pd.DataFrame({"character": ["Goku","Vegeta", "Nappa","Gohan","Piccolo"],
"power level": [12000, 16000, 4000, 1500, 3000],
"uniform color": ["orange", "blue", "black", "orange", "purple"]})
data2 = pd.DataFrame({"character": ["Gohan","Goku", "Tien","Krillin","Yamcha"],
"power level": [1500, 12000, 2000, 2000, 1500],
"unif_color": ["purple", "orange", "green", "orange", "orange"]})
data1
data2
# Use df.merge() to join data frames
data1.merge(data2,
how = "outer", # join type
on="character") # key column to join on
# Join on multiple key columns
data1.merge(data2,
how = "outer", # join type
on = ["character", "power level"]) # join on multiple cols
# Join columns with different names
# Rename columns so that they match
data2.rename(columns={'unif_color':'uniform color'}, inplace=True)
# Then join the data
data1.merge(data2,
how = "outer",
on = ["character", "power level", "uniform color"])
* Note: YouTube does not allow greater than or less than symbols in the text description, so the code above will not be exactly the same as the code shown in the video! I will use Unicode large < and > symbols in place of the standard sized ones. .
⭐ Kite is a free AI-powered coding assistant that integrates with popular editors and IDEs to give you smart code completions and docs while you’re typing. It is a cool application of machine learning that can also help you code faster! Check it out here: https://www.kite.com/get-kite/?utm_medium=referral&utm_source=youtube&utm_campaign=datadaft&utm_content=description-only