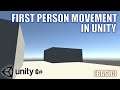
Basic First Person Character Controller in Unity - Unity C# Tutorial
In this video, I teach you guys how to make a basic first-person character controller in Unity. I've done a tutorial similar to this before, but this time instead of rotating the player with the A & D keys, you can use your mouse! This is by no means advanced and involves two simple scripts.
#Unity #Unity3D
Camera C# Script:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Cam : MonoBehaviour
{
Vector2 rotation = Vector2.zero;
public float speed = 3;
public Transform player;
void Update () {
Cursor.visible = false;
rotation.y += Input.GetAxis ("Mouse X");
rotation.x += -Input.GetAxis ("Mouse Y");
transform.eulerAngles = (Vector2)rotation * speed;
player.transform.eulerAngles = new Vector3(0, rotation.y * speed, 0);
}
}
Player Movement C# Script:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Movement : MonoBehaviour
{
public float movementSpeed;
public Rigidbody playerR;
void Update(){
if(Input.GetKey(KeyCode.W)){
playerR.velocity = transform.forward * movementSpeed * Time.deltaTime;
}
if(Input.GetKey(KeyCode.D)){
playerR.velocity = transform.right * movementSpeed * Time.deltaTime;
}
if(Input.GetKey(KeyCode.S)){
playerR.velocity = -transform.forward * movementSpeed * Time.deltaTime;
}
if(Input.GetKey(KeyCode.A)){
playerR.velocity = -transform.right * movementSpeed * Time.deltaTime;
}
}
}
If this helped you out and you want more game development Unity tutorials in the future - be sure to like, comment, share, and subscribe!
Follow me on Twitter:
https://twitter.com/omogonix
Try out my games:
https://omogonixlachlan.itch.io
Forgehub:
https://www.forgehub.com/members/omogonix.87700/
Subscribe to my Second Channel:
https://www.youtube.com/channel/UCkylkvUtpvbHfafGfIdMLBQ/featured
Join my Discord:
https://discord.gg/a88vmGD
Facebook Page:
https://www.facebook.com/Omogonix/
Follow me on Instagram:
https://www.instagram.com/uwugonixhalo/
Music Used:
Lost Sky - Vision [NCS Release]