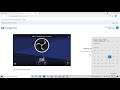
Google Meet Automation (schedule join time, turn off mic & camera)
Install selenium: Open cmd on Windows. Execute "pip install selenium"
Install Driver: https://chromedriver.chromium.org/downloads (Chrome settings, Help, About Google Chrome, Version number)
Install VS Code: https://code.visualstudio.com/download
____________________________
Source code (python)
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
from time import sleep, strftime, time,ctime
import getpass
from datetime import datetime
final_time=0
def welcome():
print('\n@@@@@@@@@@@@@@@@ Gmeet bot is ready... @@@@@@@@@@@@@@@@@@')
t=time()
print('\n /////////////// Current time : ',ctime(t)," ///////////////// \n")
def verify():
email=input("Mail address : ")
password=getpass.getpass(prompt="Password : ")
return email,password
def meet():
valid_link=1
while(valid_link):
link=input("Gmeet link : ")
link_len=len(link)
if(link_len==12):
temp_link="https://meet.google.com/"
final_link=''.join(temp_link,link)
valid_link=0
else:
final_link=link
valid_link=0
return final_link
def time_validation():
valid_time=1
while(valid_time):
final_time=int(input("\nReport time (HHMM): "))
#&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&
# change the below line as less than or equal to
if(final_time=2359):
valid_time=0
else:
print("Invalid time")
valid_time=1
return final_time
welcome()
email,password=verify()
final_link=meet()
final_time=time_validation()
opt = Options()
opt.add_argument('--disable--blink--features=AutomationControlled')
opt.add_argument('--start-maximized')
opt.add_experimental_option('prefs',{
"profile.default_content_setting_values.media_stream_mic":1,
"profile.default_content_setting_values.media_stream_camera":1,
"profile.default_content_setting_values.geolocation":0,
"profile.default_content_setting_values.notifications":1
})
driver=webdriver.Chrome(options=opt)
def login(email,password,final_link):
driver.get('https://accounts.google.com/servicelogin?hl=en&passive=true&continue=https://www.google.com/&ec=GAZAAQ')
email_id=driver.find_element_by_id('identifierId').send_keys(email)
sleep(2)
driver.find_element_by_id('identifierNext').click()
driver.implicitly_wait(100)
password_input=driver.find_elements_by_xpath('//*[@id="password"]/div[1]/div/div[1]/input')
password_input[0].send_keys(password)
sleep(5)
driver.find_element_by_id('passwordNext').click()
driver.implicitly_wait(100)
sleep(5)
driver.get(final_link)
sleep(5)
def join():
sleep(5)
driver.find_element_by_xpath('//*[@id="yDmH0d"]/c-wiz/div/div/div[9]/div[3]/div/div/div[4]/div/div/div[1]/div[1]/div/div[4]/div[1]/div/div/div').click()
driver.find_element_by_xpath('//*[@id="yDmH0d"]/c-wiz/div/div/div[9]/div[3]/div/div/div[4]/div/div/div[1]/div[1]/div/div[4]/div[2]/div/div').click()
sleep(3)
driver.find_element_by_xpath('//*[@id="yDmH0d"]/c-wiz/div/div/div[9]/div[3]/div/div/div[4]/div/div/div[2]/div/div[2]/div/div[1]/div[1]').click()
sleep(99999999999999999)
login(email,password,final_link)
while(True):
now=datetime.now()
str_time=strftime("%H%M")
current_time=int(str_time)
#&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&
#change the below line as less than or equal to
if(current_time = final_time):
break
sleep(59)
join()